ディレクトリ内のファイルを片方向同期する|Python
2021/12/23
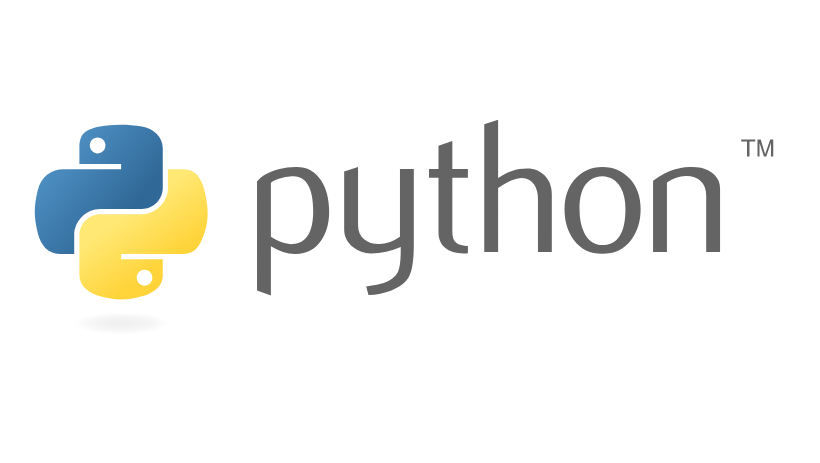
CSVで有効/無効、ターゲットのディレクトリ、宛先のディレクトリを指定し、ターゲットディレクトリのファイルを宛先のディレクトリにコピーすることで、片方向同期するスクリプトです。
例えば、ディレクトリAの中にあるファイルをすべてディレクトリBにコピーします。これをタスクスケジューラ等で定期的に実施します。ディレクトリA内のファイルが削除されても、ディレクトリBにはそのファイルは残ります。
私はとあるディレクトリの中身を片方向同期させることでファイルのバックアップをするために作成しました。
やりたいこと
簡単にやりたいことをまとめます。
- タスクスケジューラ等でスクリプトを定期実行する。
- スクリプトからCSVを読み込む。
- TargetのフォルダからDestinationのフォルダへファイルをコピーする。
CSV体系
今回利用するCSVファイルのカラムです。
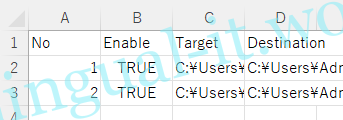
コード
コードは以下の通りです。Python初心者なのできれいか分かりませんが動きます。スクリプトのログも吐き出すようにしてます。
# v1.0 : First Version
# Instructions
# - Define parameters under No.20
# 10. Import modules
import csv
import os
import time
import shutil as sh
# 15. Functions
def Write_Log(argMsg="No message", argSeverity=3, argTimeType=1, argLogFileDirectory=None):
import os
import datetime as dt
# Parameter
DefaultLogFileDirectory = os.getcwd()
Severity = {0:"EVERGENCY",
1:"ALERT",
2:"CRITICAL",
3:"ERROR",
4:"WARNING",
5:"NOTIFICATION",
6:"INFOMATION",
7:"DEBUG"}
DatePattern = {0:"%Y/%m/%d,%H:%M:%S",
1:"%b %d %Y,%H:%M:%S",
2:"%Y:%m:%d-%H:%M:%S"}
# Get time
NowTime = dt.datetime.now()
# Create message
MessageParts = []
MessageParts += [NowTime.strftime(DatePattern[argTimeType])]
MessageParts += [Severity[argSeverity]]
MessageParts += [str(argMsg)]
Message = ",".join(MessageParts)
print(Message)
# Log file name
LogFileName = NowTime.strftime("%Y%m%d.log")
if argLogFileDirectory != None:
LogFilePath = os.path.join(argLogFileDirectory, LogFileName)
if argLogFileDirectory == None:
LogFilePath = os.path.join(DefaultLogFileDirectory, LogFileName)
# Append the message to the log file
with open(LogFilePath, "a") as LogFile:
LogFile.write(Message+"\n")
# 19. Start script
Write_Log("Starts", 6, 0)
# 20. Define parameters
Param_File = r"C:\Users\Administrator\abbr\Parameters.csv"
CSV_No = 0
CSV_Enable = 1
CSV_Target = 2
CSV_Destination = 3
# 30. Read CSV
# 30-1. Check if Parameter file exists
if os.path.exists(Param_File) == False:
Write_Log("Parameter file does not exist", 2, 0)
with open(Param_File) as Param:
reader = csv.reader(Param)
for row in reader:
# 40. Get row
# 40-1. Continue if A1 row is empty
if row[CSV_No] == "":
continue
# 40-2. Skip 1st row (where row[0] is "No")
if row[CSV_No] == "No":
continue
# 50. Get rows with parameters
if row[CSV_No] != "":
# 50-1 Skip if "Enable" is False
if row[CSV_Enable] == "False":
continue
# 50-2 Continue if target directory does not exist
if os.path.exists(row[CSV_Target]) == False:
continue
# 50-3 Get file info
for file in os.listdir(row[CSV_Target]):
Write_Log(f"Start {file}", 6, 0)
FileFullPath = os.path.join(row[CSV_Target], file)
# 50-4 Make destination directory if it doesn't exit
if os.path.exists(row[CSV_Destination]) == False:
Write_Log("Create destination dicrectory", 6, 0)
os.makedirs(row[CSV_Destination], exist_ok=True)
# 50-5 Copy target file
NewPath = os.path.join(row[CSV_Destination], file)
if os.path.exists(NewPath) == True:
Write_Log(f"file already exists,{file}")
continue
Write_Log(f"Copy,{file}", 6, 0)
Result = sh.copy(FileFullPath, row[CSV_Destination])